Auxiliary Telescope Control System (ATCS)¶
The ATCS
class groups the components that are related to Auxiliary Telescope operations, such as slewing and tracking objects.
It is built on top of the BaseTCS
.
The component CSCs that are part of this group are:
ATPtg
ATAOS
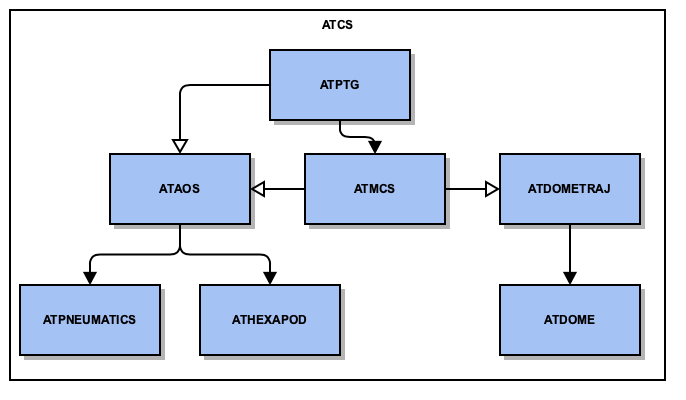
Figure 4 A hierarchical commanding architecture of the ATCS components.
The ATCS
class provides an interface to operate this group of CSCs.¶
In addition, the package also provides the ancillary class ATCSUsages
, which defines the available intended_usage
, as mentioned in Limiting Resources.
As shown in Generic CSC Group behavior the ATCS
provides both the enable
and standby
methods to facilitate setting up and shutting down.
This can be used combined with the ATCSUsages.StateTransition
usage to limit resources as needed, e.g.;
from lsst.ts.observatory.control.auxtel import ATCS, ATCSUsages
atcs = ATCS(intended_usage=ATCSUsages.StateTransition)
await atcs.start_task
# put all ATCS components in ENABLED state
await atcs.enable()
# put all ATCS components in STANDBY state
await atcs.standby()
Furthermore, the ATCS
provides prepare_for_flatfield
method to prepare the system for calibrations, which can be used with ATCSUsages.PrepareForFlatfield
(see Generic Telescope Control System (TCS) Operations).
This method will perform the following tasks subsequently;
Open the primary mirror cover. If the telescope is not in park position, it will make sure the elevation is above 70 degrees before opening the cover.
Put
ATDomeTrajectory
inDISABLED
state, to prevent it from synchronizing the telescope and the dome.Send telescope to flat-field position.
Send dome to flat-field position.
Put
ATDomeTrajectory
inENABLED
state.
Make sure the system is ENABLED
before running the task;
from lsst.ts.observatory.control.auxtel import ATCS, ATCSUsages
atcs = ATCS(intended_usage=ATCSUsages.PrepareForFlatfield)
await atcs.start_task
# put all ATCS components in ENABLED state
await atcs.enable()
# prepare ATCS for flat-field
await atcs.prepare_for_flatfield()
To prepare the telescope for on-sky activities the class provides the task prepare_for_onsky
, which can be used with ATCSUsages.StartUp
.
This method will perform the following tasks subsequently;
Slew telescope to park position (in case telescope is in flat-field position or else).
If primary mirror cover is open (e.g. for calibrations), close it. This is to ensure the mirror is protected when we start opening the dome, to avoid dust and particles from following in it.
Move dome to oppose the setting Sun, to make sure no direct sunlight hits the inside of the dome and create thermal issues.
Open dome slit.
Once dome is open, open primary mirror cover and vent gates.
Enable
ATAOS
corrections.
In general, it is advised to make sure all components are in ENABLED
state before running prepare_for_onsky
, but the method also accepts a dictionary of overrides
and calls enable
at the beginning.
from lsst.ts.observatory.control.auxtel import ATCS, ATCSUsages
atcs = ATCS(intended_usage=ATCSUsages.StartUp)
await atcs.start_task
# prepare ATCS for flat-field
await atcs.prepare_for_onsky()
Following up on what was shown in Generic CSC Group behavior, the following is also a valid way of running prepare_for_onsky
.
Overriding the overrides for a single component (e.g. ATAOS):
await atcs.prepare_for_onsky(overrides={"ataos": "constant_hex"})
Or Overriding the overrides for all components:
await atcs.prepare_for_onsky(
overrides={
"ataos": "current",
"atmcs": "",
"atptg": "",
"atpneumatics": "",
"athexapod": "current",
"atdome": "test.yaml",
"atdometrajectory": "",
}
)
It is important to remember that, if the components are already enabled, they will be left in the ENABLED
state and will not be re-cycled.
If you need to change the overrides for a specific CSC, you will have to send it to STANDBY
state first.
See Generic CSC Group behavior for an example of how to use set_state
to send individual CSCs in the group to STANDBY
state.
All the slew methods discussed in Generic Telescope Control System (TCS) Operations are available in ATCS
, which can be used with ATCSUsages.Slew
to limit resource allocation, e.g.;
from lsst.ts.observatory.control.auxtel import ATCS, ATCSUsages
atcs = ATCS(intended_usage=ATCSUsages.Slew)
await atcs.start_task
# Minimum set of parameters.
await atcs.slew_icrs(ra="00 42 44.330", dec="+41 16 07.50")
# Explicitly specify rot and target_name (both optional).
await atcs.slew_icrs(
ra="00 42 44.330", dec="+41 16 07.50", rot=0, target_name="M31"
)
# Minimum set of parameters.
await atcs.slew_object("M31")
# Explicitly specify position angle (optional).
await atcs.slew_object("M31", rot=0)
For shutting down the observatory shutdown